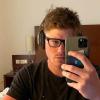
(15 items)
Many moons ago I would write web applications using technologies like PHP or Python to directly serve web content – often using templating engines to more easily handle data display. These apps would thus ship server-side rendered plain HTML, along with a mix of browser-native forms and AJAX (sometimes with JQuery) for some interactivity and more seamless data submission. I’d use additional lightly-sprinkled JavaScript for other UI niceties.
For the past ten years or so, this all changed and my web apps – including those developed as part of my work and nearly all of my side projects – switched to using front-end frameworks, like React or Vue, to create single page apps (SPAs) that instead solely consume JSON APIs. The ease of adding front-end dependencies using npm
or yarn
, advancements in modular and “importable” JavaScript, and browser-native capabilities like the Fetch API’s json()
function encouraged this further, and the ecosystem continued to grow ever larger. Front-end code would run entirely independently from the back-end, the two communicating (often) only via JSON, and with both “ends” developing their own disparate sets of logic to the extent where progressive web application front-ends could leverage service workers and run entirely offline.
For several years I’ve been using GatsbyJS to generate the static site content for this website. Gatsby is a great tool and produces blazing-fast websites through the use of an interesting combination of technologies.
In Gatsby, pages are simply React components, and developers can make use of the entire JavaScript and React ecosystems to craft their sites. Config files can be used to create pages that don’t “exist” in your filesystem (e.g. an index page for each tag used in a blog) and GraphQL queries are used to surface content and query data from across the website. Gatsby templates and standard React composition patterns allow for excellent re-use of components.
Earlier this week I needed to make some changes and re-deploy an old Vue app. I hadn’t touched the codebase in over a year, and my experience with the rate of change in the front-end web space made me dread what would happen if I tried to re-awaken this thing.
Sure enough, after running a yarn install
and launching the app using the scripts in package.json
, a number of errors were displayed about Node/Webpack/Vue incompatibilities, and I didn’t really know where to start. I don’t use Vue on a daily basis these days, and so I don’t usually need to make an effort to keep fully up-to-date on its developments, but I knew I was several versions behind on vue
, vue-loader
, as well as all the sass
and babel
toolings. This wasn’t going to be a quick fix.
Adding theming and the choice between “light” and “dark” modes to your website can enhance your site’s accessibility and make it feel more consistent with the host operating system’s own theme.
With JavaScript (and React in particular) this is easy to do, as I’ll explain in this post. We’ll use a combination of our own JavaScript code, the Zustand state management library, and the styled components package to achieve the following:
Providing code snippets on your website or blog can be a great way to convey meaning for technical concepts.
Using the HTML pre
tag can help provide structure to your listings, in terms of spacing and indentation, but highlighting keywords - as most people do in their code text editors - also vastly helps improve readability.
For example, consider the below JavaScript snippet.
class Greeter {
greet(name) {
console.log(`Hello, ${name}!`);
}
}
const greeter = new Greeter();
greeter.greet('Will');
The exact same listing is displayed below with some simple syntax highlighting. The structure and meaning of the code becomes much easier to understand.
Many web apps have support for uploading video files. Whether it’s a media-focused platform (such as a video sharing service) or just offering users a chance to add vlogs to their profile - videos are a powerful mechanism for distributing ideas.
For services providing image upload functionality, it is relatively simple to build in processes that extract smaller versions of the files (e.g. thumbnails) to be used as image previews. This allows other users to see roughly what an image is about before opening a larger version. It also enables more interesting, responsive, and attractive interfaces - since the smaller images can be loaded more quickly.
RSS has had a bit of a resurgence for personal websites and blogs in recent years, especially with the growing adoption of Small Web and IndieWeb ideologies.
Many static site generators - including Hugo, Jekyll, and Eleventy - can easily support the automatic generation of RSS feeds at build time (either directly, or through plugins).
The same is true for Gatsby - the framework currently used to build this static website - and the good news is that setting up one feed, or multiple ones for different categories, only takes a few minutes.
React state management is what gives the library its reactiveness. It’s what makes it so easy to build performant data-driven applications that dynamically update based on the underlying data. In this example the app would automatically update the calculation result as the user types in the input boxes:
import React, { useState } from 'react';
function MultiplicationCalculator() {
const [number1, setNumber1] = useState(0);
const [number2, setNumber2] = useState(0);
return ( <>
<input value={number1} onChange={e => setNumber1(parseInt(e.target.value))} />
<input value={number2} onChange={e => setNumber2(parseInt(e.target.value))} />
<p>The result is {number1 * number2}.</p>
</> );
}
If you write React web apps that interface with a backend web API then definitely consider trying React Query.
The library makes use of modern React patterns, such as hooks, to keep code concise and readable. It probably means you can keep API calls directly inside your normal component code rather than setting-up your own client-side API interface modules.
React Query will also cache resolved data through unique “query keys”, so you can keep transitions in UIs fast with cached data without needing to rely on redux.
This short post introduces a useful JavaScript operator to help make your one-liners even more concise.
The specification was added formally in the 11th edition of ECMAScript. It is implemented as a logical operator to selectively return the result of one of two expressions (or operands) based on one of the expressions resolving to a “nullish” value. A nullish value in JavaScript is one that is null
or undefined
.
JavaScript has lots of handy tools for creating concise code and one-liners. One such tool is the optional chaining operator.
The optional chaining operator is useful for addressing an attribute of a deeply-nested object in which you cannot be fully certain that the successive levels of the object are valid at run-time.
For example, consider the following object.
const person = {
name: 'Harry',
occupation: 'student',
enrolmentInformation: {
contactDetails: {
email: 'harry@hogwarts.ac.uk',
address: {
firstLine: '4 Privet Drive',
postCode: 'GU3 4GH'
}
}
}
};
In order to safely (i.e. if you cannot guarantee each object level at run-time) read the nested postCode
attribute, you could do so like this, using the logical AND operator:
Whilst working on the ITA Project - a collaborative research programme between the UK MoD and the US Army Research Laboratory - over the last few years, one of my primary areas has been to research around controlled natural languages, and working with Cardiff University and IBM UK’s Emerging Technology team to develop CENode.
As part of the project - before I joined - researchers at IBM developed the CEStore, which aims to provide tools for working with ITA Controlled English. Controlled English (CE) is a subset of the English language which is structured in a way that attempts to remove ambiguity from statements, enabling machines to understand ‘English’ inputs.
I recently wrote a new article for Heroku’s Dev Center on carrying out asynchronous direct-to-S3 uploads using Node.js.
he article is based heavily on the previous Python version, where the only major change is the method for signing the AWS request. This method was outlined in an earlier blog post.
The article is available here and there is also a companion code repository for the example it describes.
A while ago I wrote an article for Heroku’s Dev Center on carrying out direct uploads to S3 using a Python app for signing the PUT request. Specifically, the article focussed on Flask but the concept is also applicable to most other Python web frameworks.
I’ve recently had to implement something similar, but this time as part of an Node.js application. Since the only difference between the two approaches is literally just the endpoint used to return a signed request URL, I thought I’d post an update on how the endpoint could be constructed in Node.
I’ve taken to writing most of my recent presentations in plain HTML (rather than using third-party software or services). I used JavaScript to handle the appearance and ordering of slides.
I bundled the JS into a single script, js/scriptslide.js
which can be configured
using the js/config.js
script.
There is a GitHub repo for the code, along with example usage and instructions.
Most configuration can be done by using the js/config.js
script, which supports many features including: